https://school.programmers.co.kr/learn/courses/30/lessons/42576
프로그래머스
코드 중심의 개발자 채용. 스택 기반의 포지션 매칭. 프로그래머스의 개발자 맞춤형 프로필을 등록하고, 나와 기술 궁합이 잘 맞는 기업들을 매칭 받으세요.
programmers.co.kr
코드
22.04.30 첫 번째 풀이 - map 이용
#include <string>
#include <vector>
#include <map>
using namespace std;
string solution(vector<string> participant, vector<string> completion) {
map<string, int> m;
for (auto& i : participant) {
auto iter = m.find(i);
if (iter == m.end()) {
pair<string, int> p(i, 1);
m.insert(p);
}
else {
iter->second++;
}
}
for (auto& i : completion) {
map<string, int>::iterator iter = m.find(i);
(iter->second)--;
}
string answer = "";
for (auto& i : m) {
if (i.second == 1) {
answer = i.first;
}
}
return answer;
}
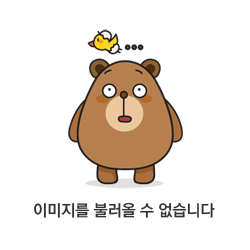
22.04.30 두 번째 풀이 - unordered_map 이용
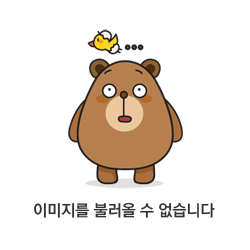
* map과 unordered_map에 대해서는 다음 글 참고.
https://chae-d-2.tistory.com/190
map, unordered_map
map 중복되지 않는 key를 가진 pair 을 담는 연관 컨테이너이다. key 값을 기준으로 sorting 되어 있고, the comparison function Compare를 사용하여 정렬된다. 대개 red-black trees를 수행한다. 이진 탐색 트리..
chae-d-2.tistory.com
'알고리즘 > Programmers' 카테고리의 다른 글
[프로그래머스/C++] 가장 큰 수 (0) | 2022.05.19 |
---|---|
[프로그래머스/C++] 더 맵게 (0) | 2022.05.19 |
[프로그래머스/C++] 기능 개발 (0) | 2022.05.19 |
[프로그래머스/C++] 전화번호 목록 (0) | 2022.05.18 |
[프로그래머스/C++] K번째 수 (0) | 2022.04.30 |